How To Use the Windows Workflow Rules Engine By Itself
Windows Workflow Foundation (WF) is a great technology for adding workflow functionality to any .NET application and it includes within it a very useful forward chaining rules engine. Because the rules engine is part of WF there is an unfortunate presumption that in order to use the rules you need to be running a workflow; but who wants to do that just to run a few rules across their objects? Especially when instantiating a workflow requires quite a bit of work or using a workflow just doesn't make sense.
Well, as it turns out you can use the workflow rules engine on it's own without any workflows at all. Because the windows workflow foundation architecture is very loosely coupled the workflow rules engine is effectively a standalone component that workflows call out to when they have rules to evaluate. We can take advantage of this from our own .NET applications and call the rules engine whenever we want a set of rules evaluated against one of our objects.
Why would we even want to do this?
The demo shows you how to create and edit sets of rules and store them in a database. Normally the rules editor is only available from within Visual Studio as part of the workflow editor but the ExternalRuleSetDemo shows how you can host the RuleSet Editor in a standard windows application and provide a backing store for the rules using an SqlExpress database.
The solution in the sample kit contains 4 projects but you really only need to look at the ExternalRuleSetLibrary project and the RuleSetTool project.
By the way, you don't have to use SqlExpress as the backing store. It's a fairly trivial process to switch it to another provider. Just go to the SaveToDB() and GetRuleSets() methods in RuleSetEditor.cs and change the code. For fun, I switched to an SqlCe Database with very little effort.
Once you've compiled the application, run it and you should see something like this (but without any Rule Sets)
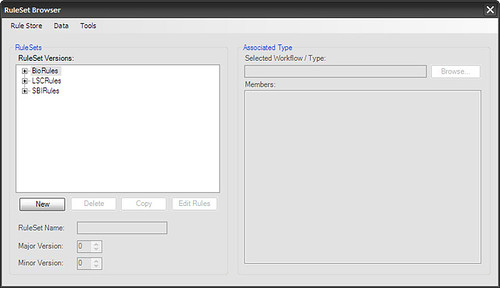
To create a rule set, click the New button. A new ruleset will be created with some default information. You can then indicate what class to base your rules on by clicking the browse button, locating the assembly that contains the class and clicking OK.
Next you can click the Edit Rules button to start the standard WF rules editor window as shown here:

Now you just need to create the rules you need and you should be ready to move to the next step (don't forget to save your rules!). There is one thing to note; you can create rules using any of the properties and methods of the target class - i.e. public, internal and private properties and methods. If you will be calling the rules engine from an assembly different to the business class then you will need to restrict the yourself to the public properties and methods or you will get runtime access permission errors.
Thankfully, the task of talking to the rules engine is actually fairly simple. What we need to do is create a helper class that will:
If you go to the samples site again you can download the RulesDrivenUI sample and have a look at the code in there. It's the basis for what's shown here.
Now, lets load up the rule set. We'll pass in a string containing the name of the rule set we want to load and then load it up from the database.
The WorkflowMarkupSerializer is used to deserialise the XML based workflow that we stored in the database (in step 1). P.S. These methods exist in a helper class, and the ruleSet variable is a private field of the RuleSet type.
GetRuleSetFromDB is shown below and once again it's pretty simple. The code show here uses the SqlCe database provider, just to prove it can be done. You can, of course, use any database mechanism you like.
Note the call to DeserializeRuleSet() [in bold]. This is where the ruleset we retrieved from the database is converted from XML back to a ruleset object. It basically wraps a call to the WorkflowMarkupSerializer as shown:
Cool! So now we've got the rules loaded up from the database.
At this point we'd love to be able to just point the rules at an object of our choice and run them, but before we do, we have to check that the rules are still valid. Why? Because when we deserialised the rules from the database we had no guarantee that those rules were still valid for the object we are going to run them against. Method signatures may have changed, properties may have been removed or we may have loaded up rules created for a completely different class. By validating the rules we protect ourselves from (most) run time errors.
To do it we use the RuleValidation class. We also use the RuleExecution class to get an execution context:
There are 2 important things happening here. Firstly we are validating the rules by passing in the type of the object we are going to run them against and checking if there are any errors. Then secondly we are creating an instance of the RuleExecution class. This is needed to store the state information used by the rule engine when validating rules. Note that while we are passing in the validated rules and the object we want to execute the rules on at this point we have not yet executed the rules, we have just created the context in which they will run.
So now we come to it. Finally! It's a really simple call to the RuleSet Execute method:
We can now wrap all these methods up into a simple method as follows:
Now to run rules for our class all we need to do is make two simple calls. Assuming we have put the above code in a helper class then we can do something like this:
Now all we need to run rules in our classes is these few lines of code.
Pretty cool, hey? If you've got any comments I'd love to hear them :-)
Well, as it turns out you can use the workflow rules engine on it's own without any workflows at all. Because the windows workflow foundation architecture is very loosely coupled the workflow rules engine is effectively a standalone component that workflows call out to when they have rules to evaluate. We can take advantage of this from our own .NET applications and call the rules engine whenever we want a set of rules evaluated against one of our objects.
Why would we even want to do this?
- Flexibility.
Why hard code business rules into your application? Every time a rules changes you need to recompile and redeploy your code. Wouldn't it be great to have all these rules available as configuration data so that all that is required to change something is to edit the rule definitions - no recompilation and no redeployment. - Ownership.When rules have to be hard coded into the application they become the property of the developers. If the rules exist as configuration data and can be modified by business people then the rules can be owned and managed by those same people, not the developers.
- Transparency.What happens when a rule isn't defined correctly or information isn't processed as expected? Normally a developer has to jump into the debugger, step through the code, find the right part of the code and check what's going on. Externalizing the rules makes it easy for non-developers to examine the rules are and see where the problem is occurring.
- Power.
It's easy enough to represent simple rules in code using if-then-else syntax, but if you've ever tried working through rule priorities or forward-chaining of rules (where one rule down the chain means a previously evaluated rule needs to be re-processed) then you know it's not an easy thing to do. The maintenance headache it represents can be daunting. By using a rules engine it becomes much easier to represent collections of rules that would be very difficult to implement in code.
Step 1: Create A Way to Enter Rules
To start with, we need some way to enter rules and somewhere to store them. And, like all good developers, we don't want to reinvent the wheel. Go to the MSDN web site; specifically the rules engine code sample and once there download and install the ExternalRuleSetDemo sample.The demo shows you how to create and edit sets of rules and store them in a database. Normally the rules editor is only available from within Visual Studio as part of the workflow editor but the ExternalRuleSetDemo shows how you can host the RuleSet Editor in a standard windows application and provide a backing store for the rules using an SqlExpress database.
The solution in the sample kit contains 4 projects but you really only need to look at the ExternalRuleSetLibrary project and the RuleSetTool project.
By the way, you don't have to use SqlExpress as the backing store. It's a fairly trivial process to switch it to another provider. Just go to the SaveToDB() and GetRuleSets() methods in RuleSetEditor.cs and change the code. For fun, I switched to an SqlCe Database with very little effort.
Once you've compiled the application, run it and you should see something like this (but without any Rule Sets)
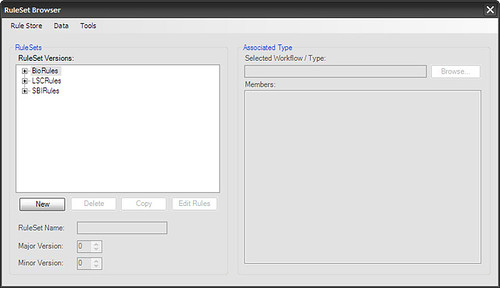
To create a rule set, click the New button. A new ruleset will be created with some default information. You can then indicate what class to base your rules on by clicking the browse button, locating the assembly that contains the class and clicking OK.
Next you can click the Edit Rules button to start the standard WF rules editor window as shown here:

Now you just need to create the rules you need and you should be ready to move to the next step (don't forget to save your rules!). There is one thing to note; you can create rules using any of the properties and methods of the target class - i.e. public, internal and private properties and methods. If you will be calling the rules engine from an assembly different to the business class then you will need to restrict the yourself to the public properties and methods or you will get runtime access permission errors.
Step 2: Calling the Rules Engine
Ok, so now we have a way to create and edit rules. That's great, but pretty much useless unless we have an application in which to use those rules.Thankfully, the task of talking to the rules engine is actually fairly simple. What we need to do is create a helper class that will:
- Retrieve (and deserialize) the rules from the database.
- Validate the rules against the class we want to run them against
- Execute them against the object we choose.
If you go to the samples site again you can download the RulesDrivenUI sample and have a look at the code in there. It's the basis for what's shown here.
Now, lets load up the rule set. We'll pass in a string containing the name of the rule set we want to load and then load it up from the database.
public void LoadRuleSet(string ruleSetName)
{
WorkflowMarkupSerializer serializer = new WorkflowMarkupSerializer();
if (string.IsNullOrEmpty(ruleSetName))
throw new Exception("Ruleset name cannot be null or empty.");
if (!string.Equals(RuleSetName, ruleSetName))
{
_ruleSetName = ruleSetName;
ruleSet = GetRuleSetFromDB(serializer);
if (ruleSet == null)
{
throw new Exception("RuleSet could not be loaded. Make sure the connection string and ruleset name are correct.");
}
}
}
The WorkflowMarkupSerializer is used to deserialise the XML based workflow that we stored in the database (in step 1). P.S. These methods exist in a helper class, and the ruleSet variable is a private field of the RuleSet type.
GetRuleSetFromDB is shown below and once again it's pretty simple. The code show here uses the SqlCe database provider, just to prove it can be done. You can, of course, use any database mechanism you like.
private RuleSet GetRuleSetFromDB(WorkflowMarkupSerializer serializer)
{
if (string.IsNullOrEmpty(_connectionString))
return null;
SqlCeDataReader reader;
SqlCeConnection sqlConn = null;
try {
sqlConn = new SqlCeConnection(_connectionString);
sqlConn.Open();
SqlCeParameter p1 = new SqlCeParameter("@p1",_ruleSetName);
string commandString = "SELECT * FROM RuleSet WHERE Name= @p1 ORDER BY ModifiedDate DESC";
SqlCeCommand command = new SqlCeCommand(commandString, sqlConn);
command.Parameters.Add(p1);
reader = command.ExecuteReader();
}
catch (Exception e)
{
...
}
RuleSet resultRuleSet = null;
try {
reader.Read();
resultRuleSet = DeserializeRuleSet(reader.GetString(3), serializer);
}
catch {
...
}
sqlConn.Close();
sqlConn.Dispose();
return resultRuleSet;
}
Note the call to DeserializeRuleSet() [in bold]. This is where the ruleset we retrieved from the database is converted from XML back to a ruleset object. It basically wraps a call to the WorkflowMarkupSerializer as shown:
StringReader stringReader = new StringReader(ruleSetXmlDefinition);
XmlTextReader reader = new XmlTextReader(stringReader);
return serializer.Deserialize(reader) as RuleSet;
Cool! So now we've got the rules loaded up from the database.
At this point we'd love to be able to just point the rules at an object of our choice and run them, but before we do, we have to check that the rules are still valid. Why? Because when we deserialised the rules from the database we had no guarantee that those rules were still valid for the object we are going to run them against. Method signatures may have changed, properties may have been removed or we may have loaded up rules created for a completely different class. By validating the rules we protect ourselves from (most) run time errors.
To do it we use the RuleValidation class. We also use the RuleExecution class to get an execution context:
private RuleExecution ValidateRuleSet(object targetObject)
{
RuleValidation ruleValidation;
ruleValidation = new RuleValidation(_targetType, null);
if (!ruleSet.Validate(ruleValidation))
{
string errors = "";
foreach (ValidationError validationError in ruleValidation.Errors)
errors = errors + validationError.ErrorText + "\n";
Debug.WriteLine("Validation Errors \n" + errors);
return null;
}
else {
return new RuleExecution(ruleValidation, targetObject);
}
}
There are 2 important things happening here. Firstly we are validating the rules by passing in the type of the object we are going to run them against and checking if there are any errors. Then secondly we are creating an instance of the RuleExecution class. This is needed to store the state information used by the rule engine when validating rules. Note that while we are passing in the validated rules and the object we want to execute the rules on at this point we have not yet executed the rules, we have just created the context in which they will run.
So now we come to it. Finally! It's a really simple call to the RuleSet Execute method:
private void ExecuteRule(RuleExecution ruleExecution)
{
if (null != ruleExecution)
{
ruleSet.Execute(ruleExecution);
}
else {
throw new Exception("RuleExecution is null.");
}
}
We can now wrap all these methods up into a simple method as follows:
public void ExecuteRuleSet(object targetObject)
{
if (ruleSet != null)
{
RuleExecution ruleExecution;
ruleExecution = ValidateRuleSet(targetObject);
ExecuteRule(ruleExecution);
}
}
Now to run rules for our class all we need to do is make two simple calls. Assuming we have put the above code in a helper class then we can do something like this:
WorkflowRulesHelper wfhelper = new WorkflowRulesHelper();
wfhelper.LoadRuleSet("LSCRules");
wfhelper.ExecuteRuleSet(this);
Now all we need to run rules in our classes is these few lines of code.
Pretty cool, hey? If you've got any comments I'd love to hear them :-)